1. 아래를 참고하여 출력값이 나오도록 프로그래밍 하시오.
· 참고
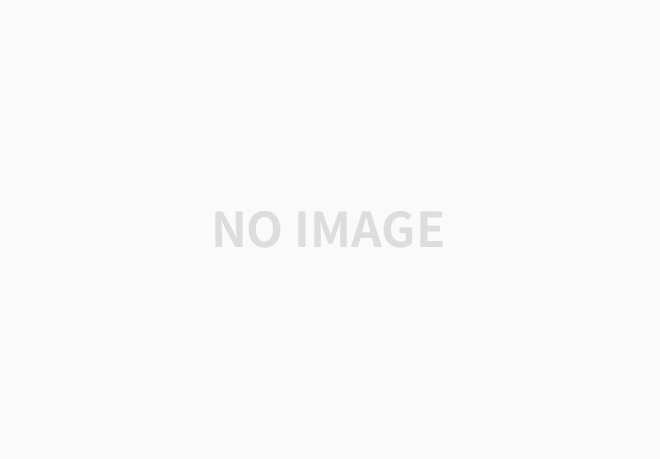
· 작성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
class DBox <F, N> {
private F fruit;
private N num;
public void set(F f, N n) {
fruit = f;
num = n;
}
@Override
public String toString() {
return fruit + " & " + num;
}
}
class DDBox<F2, N2>{
private F2 fruit2;
private N2 num2;
public void set(F2 f2, N2 n2) {
fruit2 = f2;
num2 = n2;
}
@Override
public String toString() {
super.toString();
return fruit2 + "\n" + num2;
}
}
|
cs |
2. 아래를 참고하여 출력값이 나오도록 프로그래밍 하시오.
· 참고
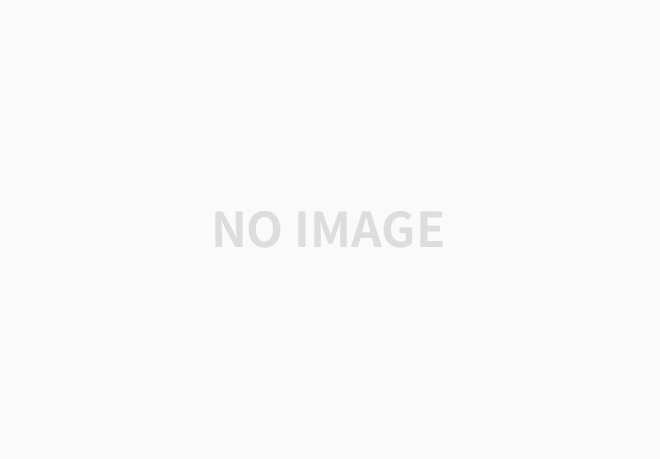
· 작성
1
2
3
4
5
6
7
8
9
10
|
class Box<T> {
private T ob;
public void set(T o) {
ob = o;
}
public T get() {
return ob;
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
class Boxmain {
public static void main(String[] args) {
Box<Integer> box1 = new Box<>();
box1.set(99);
Box<Integer> box2 = new Box<>();
box2.set(55);
System.out.println(box1.get() + " & " + box2.get());
swapBox(box1, box2);
System.out.println(box1.get() + " & " + box2.get());
}
public static void swapBox(Box<Integer> box1, Box<Integer> box2) {
int temp = 0;
temp = box1.get();
box1.set(box2.get());
box2.set(temp);
}
}
|
cs |
3. 아래를 참고하여 출력값이 나오도록 프로그래밍 하시오.
· 참고
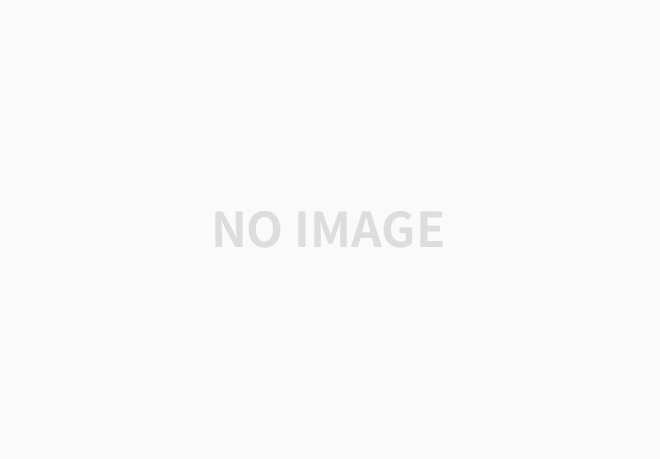
· 작성
1
2
3
4
5
6
7
8
9
10
|
class Box<T> {
private T ob;
public void set(T o) {
ob = o;
}
public T get() {
return ob;
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
|
class Boxmain {
public static void main(String[] args) {
Box<Integer> box1 = new Box<>();
box1.set(24);
Box<String> box2 = new Box<>();
box2.set("Poly");
if(compBox(box1, 25))
System.out.println("상자 안에 25 저장");
if(compBox(box2, "Moly"))
System.out.println("상자 안에 Moly 저장");
System.out.println(box1.get());
System.out.println(box2.get());
}
private static boolean compBox(Box<Integer> box1, int num) {
if(box1.get() == num)
return true;
else
return false;
}
private static boolean compBox(Box<String> box2, String str) {
if(box2.get() == str)
return true;
else
return false;
}
}
|
cs |
'bitcamp > JAVA' 카테고리의 다른 글
컬렉션 프레임워크_연습문제1 (0) | 2021.02.06 |
---|---|
컬렉션 프레임워크 (0) | 2021.02.05 |
제네릭 (0) | 2021.01.25 |
자바의 기본 class_연습문제 (0) | 2021.01.25 |
자바의 기본 class (0) | 2021.01.25 |