1. 아래의 결과 값은 false 출력이 된다. true가 되도록 INum을 작성하시오.
· 참고
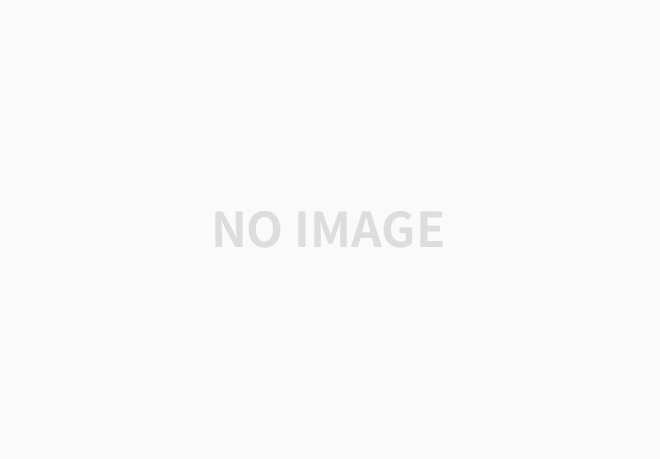
· 작성 : 참고에서 Arrays equals(ar1, ar2)는 객체를 비교하기 때문에 서로 다른 인스턴스를 참조하고 있어 false를 출력한다.
작성한 INum에서는 equals 함수 오버라이딩을 하여 단순히 내용을 비교하기 때문에 true를 출력하게 된다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
class INum{
private int num;
public INum(int num){
this.num = num;
}
@Override
public boolean equals(Object obj){
if(this.num == ((INum)obj).num)
return true;
else
return false;
}
public static void main(String[] args) {
INum[] ar1 = new INum[3];
INum[] ar2 = new INum[3];
ar1[0] = new INum(1); ar2[0] = new INum(1);
ar1[1] = new INum(2); ar2[1] = new INum(2);
ar1[2] = new INum(3); ar2[2] = new INum(3);
System.out.println(Arrays.equals(ar1, ar2));
}
}
|
cs |
2. 아래의 내용을 참고하여 이름순으로 정렬되게 Person 객체를 작성하시오.
· 참고
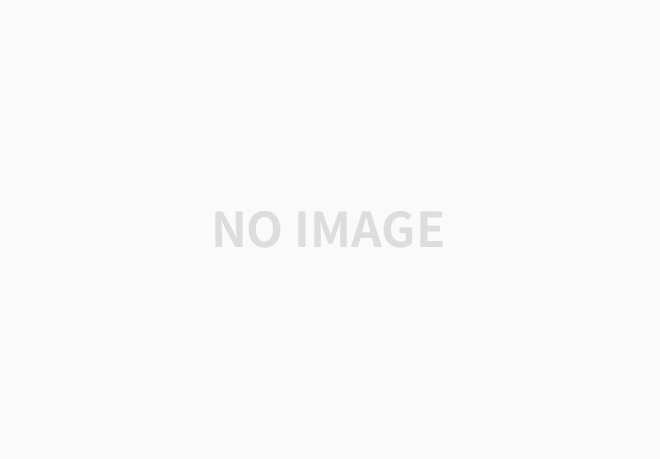
· 작성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
class Person implements Comparable{
private String name;
private int age;
public Person(String name, int age){
this.name = name;
this.age = age;
}
@Override
public int compareTo(Object o){
Person p = (Person)o;
return this.name.compareTo((p.name).name);
}
@Override
public String toString() {
return name + ": " + age;
}
}
|
cs |
3. 위의 문제에서 이름 글자수가 많은 순으로 정렬되게 person 객체를 작성하시오.
· 작성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
class Person2 implements Comparable{
private String name;
private int age;
public Person2(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public int compareTo(Object o){
Person2 p = (Person2)o;
return p.name.length() - this.name.length();
}
@Override
public String toString() {
return name + ": " + age;
}
}
|
cs |
4. 아래를 프로그래밍 하시오.
· 참고
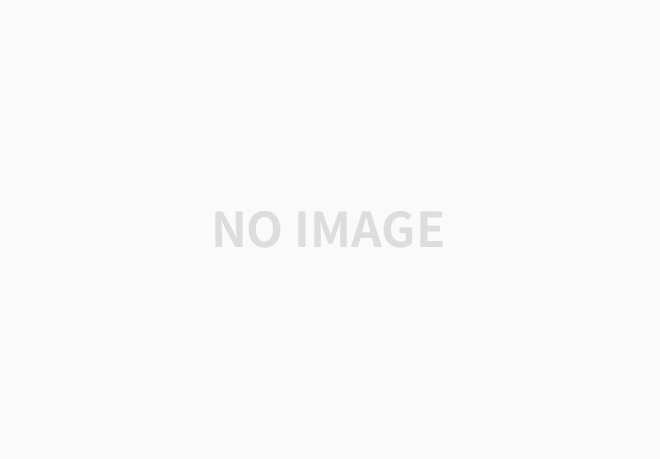
· 작성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
class Rectangle implements Comparable{
private int width;
private int heigth;
public Rectangle(int width, int heigth) {
this.width = width;
this.heigth = heigth;
}
public int getArea() {
return width * heigth;
}
@Override
public int compareTo(Object o) {
return this.getArea() - ((Rectangle2)o).getArea();
}
public String toString() {
return this.getArea() + ", ";
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
class Rectanglemain{
public static void main(String[] args) {
Scanner sc =new Scanner(System.in);
Rectangle[] rec = new Rectangle[4];
for(int i = 0; i < rec.length; i++) {
int w = sc.nextInt();
int h = sc.nextInt();
rec[i] = new Rectangle(w, h);
}
Arrays.sort(rec);
for(Rectangle e : rec)
System.out.print(e);
System.out.println();
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
class Rec {
public static Rectangle[] getSortingRec(Rectangle[] recArr) {
Rectangle[] rec = new Rectangle[4];
Rectangle temp = null;
if (rec instanceof Comparable[]) {
for (int i = 0; i < rec.length; i++) {
for (int j = 0; j < rec.length - i - 1; j++) {
if (rec[j].compareTo(rec[j + 1]) > 0) {
temp = rec[j];
rec[j] = rec[j + 1];
rec[j + 1] = temp;
}
}
}
} else {
for (int i = 0; i < rec.length; i++) {
for (int j = 0; j < rec.length - i - 1; j++) {
if (rec[j].getArea() > rec[j + 1].getArea()) {
temp = rec[j];
rec[j] = rec[j + 1];
rec[j + 1] = temp;
}
}
}
}
return rec;
}
}
|
cs |
5. 다음 조건을 만족하도록 오늘의 정보를 출력하는 프로그램을 작성하시오.
· 참고: Calendar 객체의 다음 메소드를 사용할 것!
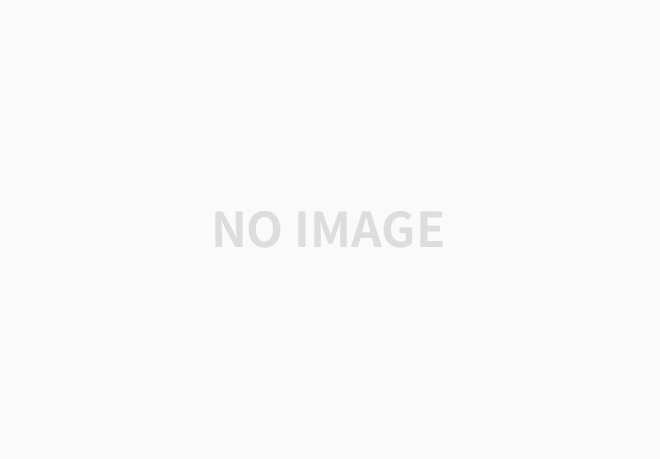
· 작성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
|
class Calendarmain {
public static void main(String[] args) {
Calendar cal = Calendar.getInstance();
int year = cal.get(Calendar.YEAR);
int mon = cal.get(Calendar.MONTH);
int day = cal.get(Calendar.DATE);
int week = cal.get(Calendar.DAY_OF_WEEK);
String str = "a";
switch(week) {
case (1) :
str = "일";
break;
case (2) :
str = "월";
break;
case (3) :
str = "화";
break;
case (4) :
str = "수";
break;
case (5) :
str = "목";
break;
case (6) :
str = "금";
break;
case (7) :
str = "토";
break;
}
System.out.println("오늘은 " + year + "년 " + (mon+1) + "월 " + day + "일 " + str + "요일입니다.");
System.out.println("이 달의 " + cal.get(Calendar.DAY_OF_WEEK_IN_MONTH) + "번째 " + str + "요일입니다.");
System.out.println("이 달의 " + cal.get(Calendar.WEEK_OF_MONTH) + "번째 주입니다.");
System.out.println("이 해의 " + cal.get(Calendar.DAY_OF_YEAR) + "일입니다.");
System.out.println("이 해의 " + cal.get(Calendar.WEEK_OF_YEAR) + "번째 주입니다.");
}
}
|
cs |
6. 다음 예시를 참고하여 경과시간을 맞추는 게임을 작성하시오.
· 참고: <Enter>키를 입력하면 현재 초 시간을 보여주고
여기서 10초에 더 근접하도록 다음 <Enter> 키를 입력한 사람이 이기는 게임이다.
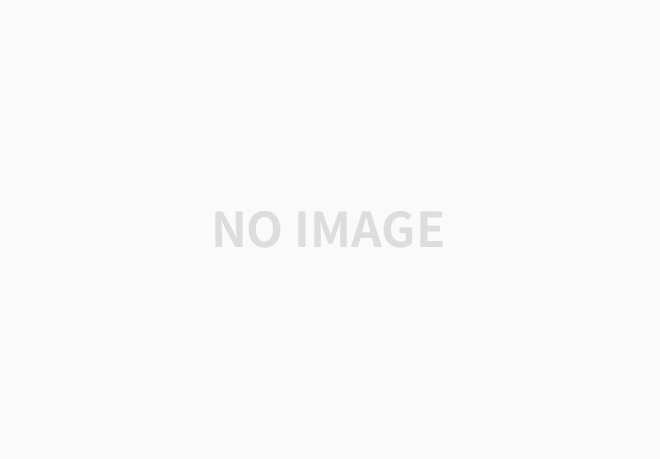
· 작성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
class Game {
private String name;
private int result;
Game(String name){
this.name = name;
}
@Override
public String toString() {
return name;
}
public void getPlay() {
Scanner sc = new Scanner(System.in);
System.out.print(name + " 시작 키 >>");
sc.nextLine();
Calendar cal = Calendar.getInstance();
int sec = cal.get(Calendar.SECOND);
System.out.println(" 현재 초 시간 = " + sec);
System.out.print("10초 예상 후 키 >> ");
sc.nextLine();
Calendar cal2 = Calendar.getInstance();
int sec2 = cal2.get(Calendar.SECOND);
System.out.println(" 현재 초 시간 = " + sec2);
if(sec2 >= sec) {
result = sec2 - sec;
}else{
result = (sec2 + 60) - sec;
}
public int getResult() {
return result;
}
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
class Gamemain {
public static void main(String[] args) {
System.out.println("10초에 가까운 사람이 이기는 게임입니다.");
Game user1 = new Game("황기태");
Game user2 = new Game("이재문");
user1.getPlay();
user2.getPlay();
System.out.println("황기태의 결과" + user1.getResult() + ", 이재문의 결과" + user2.getResult());
if(user1.getResult() > user2.getResult()) {
System.out.println("승자는 " + user2);
}else if(user1.getResult() < user2.getResult()){
System.out.println("승자는 " + user1);
}else{
System.out.println("무승부입니다.");
}
}
}
|
cs |
7. 아래를 프로그래밍 하시오.
· 참고
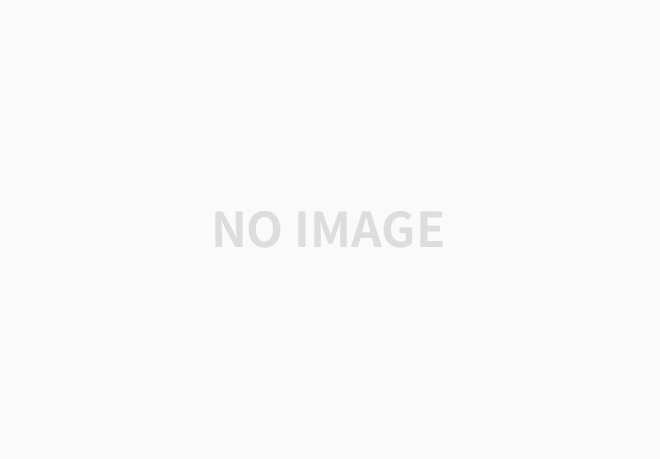
· 작성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
class Rectangle{
private int width;
private int heigth;
public Rectangle(int width, int heigth) {
this.width = width;
this.heigth = heigth;
}
public int getHeight() {
return heigth;
}
public void setHeight() {
this.heigth = heigth;
}
public int getWidth() {
return width;
}
public void setWeight() {
this.width = width;
}
public static Rectangle compareRect(Rectangle r1, Rectangle r2) {
if((r1.width < r2.width) && (r1.heigth < r2.heigth)){
return r2;
}else
return r1;
}
}
|
cs |
'bitcamp > JAVA' 카테고리의 다른 글
제네릭_연습문제 (0) | 2021.01.26 |
---|---|
제네릭 (0) | 2021.01.25 |
자바의 기본 class (0) | 2021.01.25 |
Object 클래스_연습문제 (0) | 2021.01.25 |
JVM 메모리모델과 Object 클래스 (0) | 2021.01.25 |